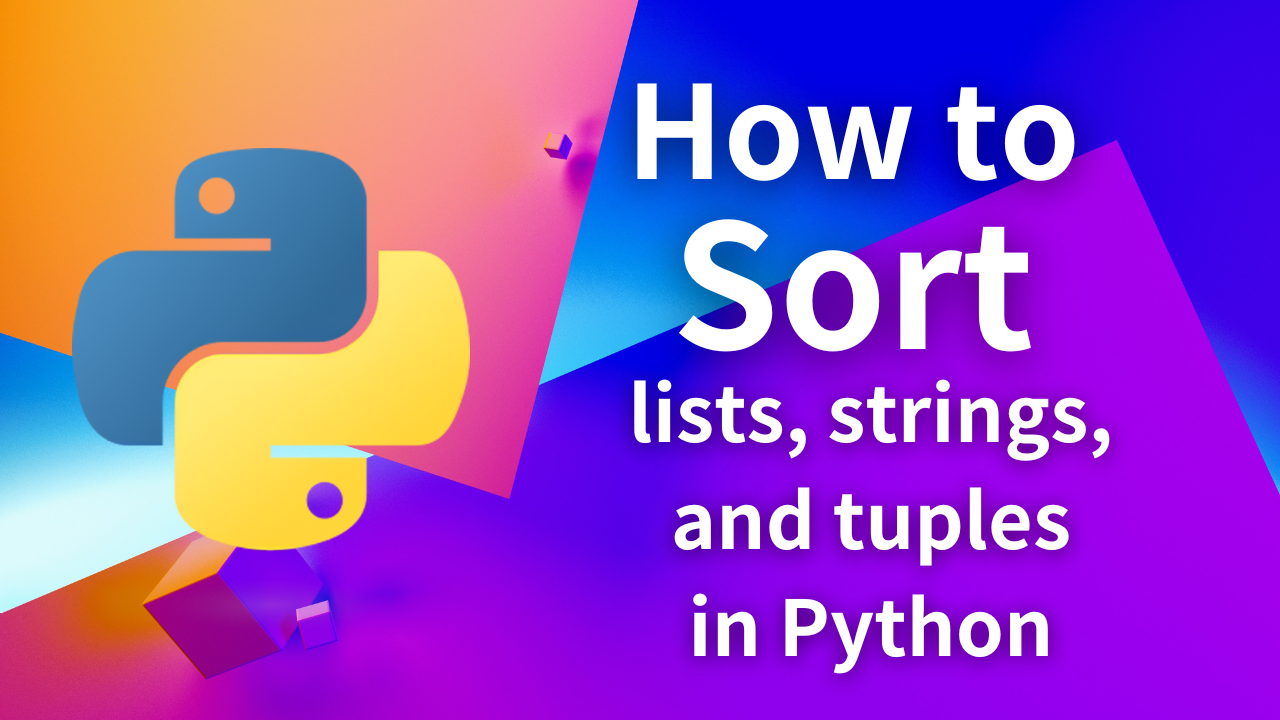
How to sort lists, tuples, and strings in Python
In this guide, you will learn how to use Python’s sort()
method and sorted()
function to sort data sets. Sorting is an essential skill to become a professional programmer, and by the end of this article, you will be able to use this skill with ease.
Easiest way to sort (Method #1)
The simplest way to sort items in Python is using the sort
method. Sorting with the sort
method can be done like the code below.
numbers = [0,100,20,30,40,80,10]
numbers.sort()
print(numbers)
## Output: [0, 10, 20, 30, 40, 80, 100]
As you can see, the sort
method quickly sorts any list in order. Now let’s get into the argument that you could include to use in other situations like you want to sort from largest to smallest. You can do that by using the reverse
argument. This option will reverse the order of sorting from largest to smallest.
The reverse argument can be used like the code below:
numbers = [0,100,20,30,40,80,10]
numbers.sort(reverse=True)
print(numbers)
## Output: [100, 80, 40, 30, 20, 10, 0]
To sort a list with strings, you can use the key
argument. For example, the code below sorts the list of fruit in alphabetical order.
Fruits = ["Banana", "Apple", "Lemon"]
Fruits.sort(key=str.lower)
print(Fruits)
# Output => Apple Banana Lemon
The value str.lower
converts the string value to lowercase.
With these examples now you understand how you can sort a list in Python. Yet, the sort()
method only accepts a list as a data type.
Then how should you sort other types of data structures like strings?
This is where the second method sorted()
function comes in handy.
The alternative: Method #2 Sorted()
The sorted function is similar to the sort function except for its uses and how it doesn’t destroy the original variable. Particularly, if you use the sort()
function, it destroys the original list. This means that you will not be able to keep the original values in your list.
One example of the way you can use the sorted function is like the code below:
numbers = [0,100,20,30,40,80,10]
sortednumbers = sorted(numbers)
print(sortednumbers)
# The output: [100, 80, 40, 30, 20, 10, 0]
As mentioned earlier, you can use the sorted()
function to sort other data types like tuples and strings.
For the tuple type variable, you can use the sorted()
function in the same way as the list. For instance, you can sort your tuple in order using several lines of code like the example below:
tupleA = (10,10,20,30,50,9,1,2,8)
print(sorted(tupleA))
## Result: [1, 2, 8, 9, 10, 10, 20, 30, 50]
While it successfully arranged the values of a tuple variable in order, however, the sorted value is transferred into a list when it is sorted. To fix this issue and keep the variable in the tuple data type, you can use the tuple()
function to convert the result back to the original tuple data type. This can be done in the upgraded version of the previous code:
tupleA = (10,10,20,30,50,9,1,2,8)
print(tuple(sorted(tupleA)))
## Result: (1, 2, 8, 9, 10, 10, 20, 30, 50)
Now let’s move into how you can sort a string variable. Similar to how you can sort a tuple, you can sort a string by simply using the sort()
function. By using the code shown below, you can sort a String into A-Z order:
Apple = "Apple"
print(sorted(Apple))
#Result: ['A', 'e', 'l', 'p', 'p']
Conclusion
In this article, we learned that:
- You can use
sort()
method to quickly sort a list. - You can use the
sorted()
function to sort most datatypes.
Before ending this article, I want to note that supporting my blog through social media and linking to your website will be very helpful. Thank you for reading.
Image Attribution
Photo by Milad Fakurian on Unsplash