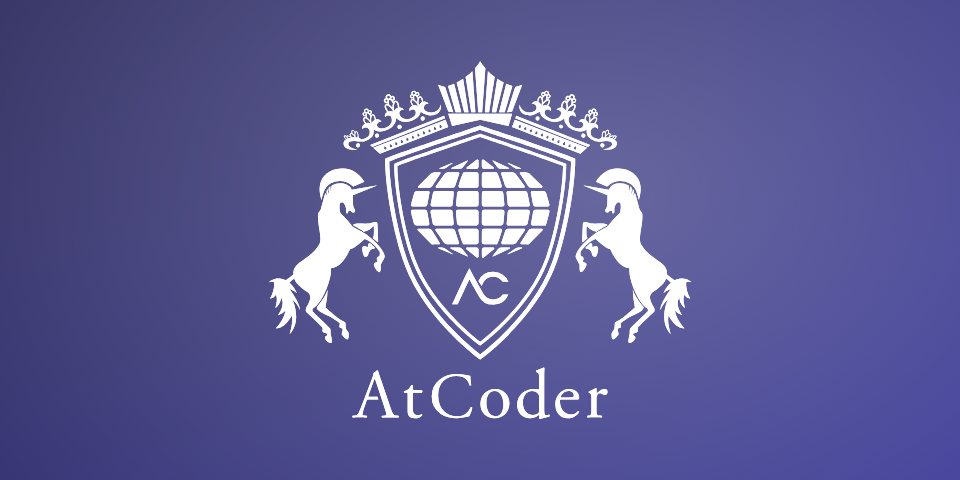
Atcoder Beginners Contest 350 Recap
How did you do in Atcoder Beginners Contest 350? For me, I got A and B correct. My performance score was 362! This guide will explain how I solved A through B during the competition.
Problem A “Past ABCs”
Problem:
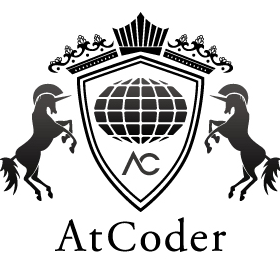
Guide
The problem A in this contest was somewhat easy. Probably this was the problem that most people got AC since in Atcoder Problems it is rated 32. The only thing you have to do is to check if it’s a valid contest name that existed or not. This can be done by putting an if statement like this:
if S != "ABC316" and int(S[3:6]) <= 349 and int(S[3:6]) > 0 and "ABC" in S and len(S) == 6:
Other than that, the only thing you have to be careful about is some exceptions. For instance, ABC350
and ABC316
are not a valid contest name.
So, the code prints “Yes” if it meets all the requirements and prints “No” if it doesn’t. It is as simple as the code below.
# -*- coding: utf-8 -*-
S = input()
if S != "ABC316" and int(S[3:6]) <= 349 and int(S[3:6]) > 0 and "ABC" in S and len(S) == 6:
print("Yes")
else:
print("No")
Link to my submission (As a proof):
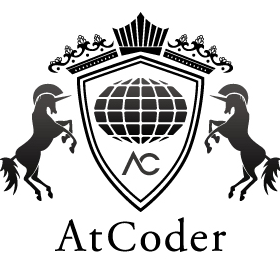
Problem B “Dentist Aoki”
Next, I would like to discuss problem B. In my opinion, problem B was much easier to understand and solve than problem A. Let’s take a look at the guide.
Problem
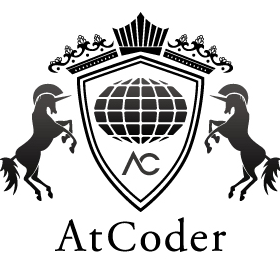
Guide
The way to solve Problem B is fairly simple. You have to create a list named teeth
which holds either “0” or “1” depending on whether he has teeth in a specific position or not.
First, we get the input from the problem using the code below.
N, Q = map(int,input().split())
Ts = list(map(int,input().split()))
Then when the teeth[i] is 0, the code changes the value to 1. And when it is the opposite, Teeth[i] changes to 1.
if Teeth[number] == 0:
Teeth[number] = 1
After all the treatment is done (when the for loop finishes), the code prints the number 1 in the list.
print(Teeth.count(1))
Here is my code that got AC on this problem.
Code
# -*- coding: utf-8 -*-
N, Q = map(int,input().split())
Ts = list(map(int,input().split()))
Teeth = []
for i in range(0,N):
Teeth.append(1)
for i in range(0,Q):
number = Ts[i]-1
if Teeth[number] == 0:
Teeth[number] = 1
continue
if Teeth[number] == 1:
Teeth[number] = 0
continue
print(Teeth.count(1))
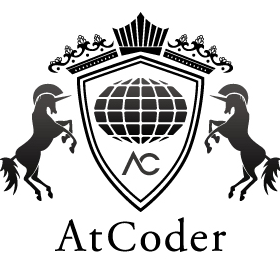
Conclusion
In conclusion, Atcoder Beginner’s Contest 350 was another awesome contest with lots of great problems and ways to solve them. There are millions of possibilities to solve each problem, so I recommend you look for other people’s submissions in your language that are correct if you want to dig deep into other ways of solving these problems. I hope someone finds this article useful. Please consider sharing this page on your social media so you can support this blog. Thank you for reading.