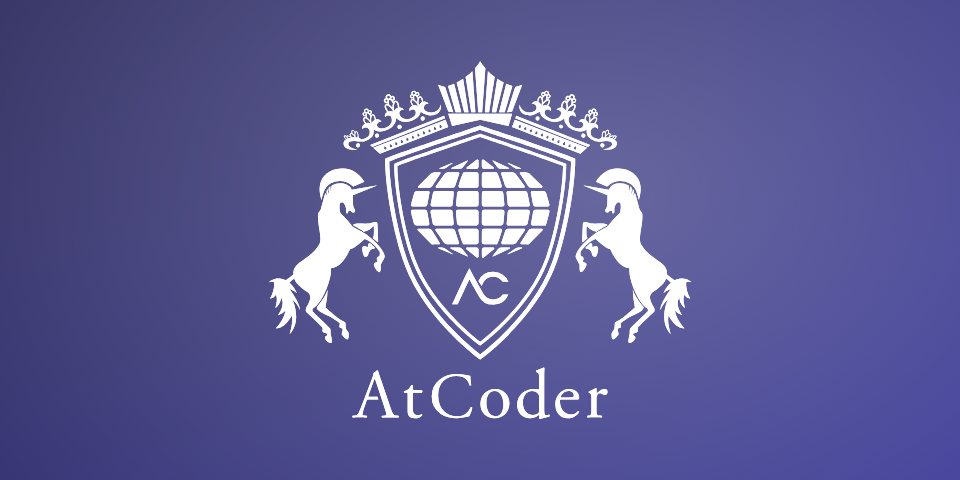
Atcoder Beginners Contest 349 Recap
How did you do in Atcoder Beginners Contest 349? For me, I got A and B correct and I gained AC on C after the competition. This guide will explain how I solved A through B during the competition, and how I got C after it. Let’s dive in!
Problem A “Zero Sum Game”
Problem:
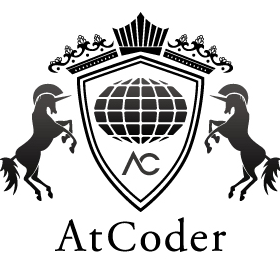
Guide
The problem A in this contest was somewhat convoluted. This is because to solve this question, you had to deeply understand what the problem was asking for and how to calculate it. Yet, it becomes so simple once you understand the fact that the order of the game doesn’t affect the result. , I didn’t understand this and I got stuck on this problem for a while until I finally noticed that it was a question that asked me to make a simple process to calculate the player’s unknown points by calculating the number of wins and losses for other players.
The way I used to solve this problem is simple. I thought of the array As as a list with each person’s number of victories. Then, I checked if the person[i] has won a lot or not (A>0), and if so delete the expected score by the score of person[i]. This goes for the opposite. If a person[i]‘s score is negative, then I added their score to the expected score. And finally, I print out the expected score, and the code finish.
Here is a code that I got AC on this problem:
# -*- coding: utf-8 -*-
N = int(input())
As = list(map(int,input().split()))
answer = 0
for i in range(0,len(As)):
if As[i] > 0:
answer -= As[i]
continue
elif As[i] < 0:
answer += abs(As[i])
continue
print(answer)
Link to my submission (As a proof):
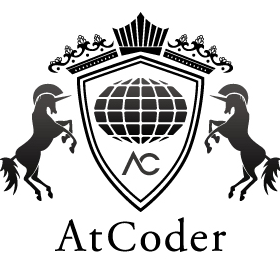
Problem B “Commencement”
Next, I would like to discuss problem B. In my opinion, problem B was much easier to understand and solve than problem A. Let’s take a look at the guide.
Problem
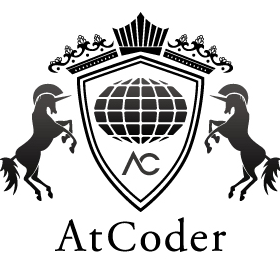
Guide
The way to solve Problem B is fairly simple. You have to create a list of letters
with each letter’s frequency and count the number of letters with the same frequency in the array letters
. If all the number of letters with the same frequency is either 2 or 0, then the code prints Yes. If not, the code will print No.
To give more explanation on what I just said, let’s take a look at the printed output of the list of “letters” in my code.
commencement
[2, 1, 3, 3, 2, 1]
As you can see, the list contains the frequency of each letter in a given word, starting with the frequency of ‘c’ and ending with the frequency of ‘t’. We can use this list inside a for loop to count the number of a number in the list. The code below is an example of a for loop that does this job perfectly.
for i in range(0,len(letters)):
if letters.count(letters[i]) == 2:
continue
elif letters.count(letters[i]) == 0:
continue
else:
print("No")
exit()
For each turn, the code calculates the frequency of a specific letter in Letters[i] using the .count()
method. If the returned value is either two or zero, the for loop continues; if the value is neither one of them, it stops the code by printing no.
Here is my code that got AC on this problem.
Code
# -*- coding: utf-8 -*-
S = input()
letters = []
alreadyused = []
for i in range(0,len(S)):
if S[i] not in alreadyused:
letters.append(S.count(S[i]))
alreadyused.append(S[i])
continue
else:
continue
for i in range(0,len(letters)):
if letters.count(letters[i]) == 2:
continue
elif letters.count(letters[i]) == 0:
continue
else:
print("No")
exit()
print("Yes")
exit()
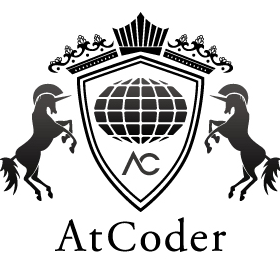
Problem C “Airport code”
Finally, I would like to explain problem C “Airport code.” This problem is one of my favorite C problems in Atcoder because of how this problem is really straightforward and also delightful. The link to the problem is attached below.
Problem
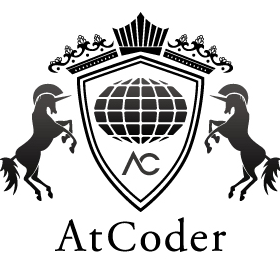
Guide
This problem is one of the simplest problems in the world. It gives you two values, S which tells the airport’s name, and T which gives the airport’s code. The problem asks one simple question: Is the given airport code a valid airport code? According to the problem, a valid airport code satisfies either of these two requirements.
- The code is a capitalized version of the substring(it can have some distance- like Narita’s code can be NRA).
- The first two letters of the code are the capitalized version of a substring of
S
, and the third letter is X.
Now that we know what the question asks, it gets to “how?”
The “how” part can be done in multiple ways. The way I and a user from whom I got my code inspired was to run a for loop that checks for each letter in T
with S
. If it all matches three times or it matches two times but the third letter in T is “X”, the code prints “Yes”, and if this is not true then the code prints “No”.
Here is my version of this algorithm in code. Feel free to tweak some parts to understand how it works in detail.
S = input().upper()
T = input()
# Count=> The number of letters of T so that is in the airport's name
Count = 0
for i in S:
if T[Count] == i:
Count+=1
if Count == 3:
print("Yes")
exit()
elif Count == 2 and T[2] == "X":
print("Yes")
exit()
else:
continue
else:
continue
# If not all the letters in T were in the airport's name, then print no.
print("No")
exit()
Conclusion
In conclusion, Atcoder beginner’s Contest 349 was another superb contest with lots of great problems and ways to solve them. There are millions of possibilities to solve each problem, so I recommend you look for other people’s submissions in your language that are correct if you want to dig deep into other ways of solving these problems. I will be thrilled if someone finds this article useful. Thank you for reading.